백고등어 개발 블로그
typeorm 연결(with mysql)과 사용 본문
우선, typeorm 설치와 설정이 모두 끝난 상태라는 가정하에 typeorm 을 통해 mysql 연동을 해보겠습니다.
설치와 설정에 관련된 내용은 아래를 참고해주세요!
TypeScript와 Typeorm을 사용해express 프로젝트 세팅
npm init : package.json 파일 생성 npm install -g typescript : grobal로 typescript 설치, 처음 프로젝트에서만 설치하면 된다. tsc --init : tsconfig.json 파일 생성 tsconfic.json 파일
velog.io
1. typeorm 을 통한 mysql 연결
typeorm 을 통해 mysql 을 연결하기 위해선 typeorm 의 createConnection 메소드를 사용하시면 됩니다
아래의 코드를 참고해주세요!
🎯 createConnection 메소드는 자동으로 ormconfig.json 찾아서 연결 옵션들을 읽습니다.
더 자세한 내용은 아래의 링크를 참고해주세요!
TypeORM - Amazing ORM for TypeScript and JavaScript (ES7, ES6, ES5). Supports MySQL, PostgreSQL, MariaDB, SQLite, MS SQL Server,
typeorm.io
- index.ts (전체 코드중 연결코드만)
// typeorm 모듈의 createConnection 메소드를 가져옵니다.
import { createConnection } from "typeorm";
// createConnection 메소드는 Promise 객체를 반환하므로 아래와 같이 작성하였습니다.
// 그리고 then 메소드안에 서버 실행 코드를 작성한 이유는
// 데이터베이스 연결을 먼저한 후 서버를 실행시켜야 원활하게 동작할 것이기 때문입니다.
// 물론 데이터베이스 연결을 무조건 먼저하지 않아도 되지만
// 데이터베이스 연결이 우선이 되는 기능을 구현하실 땐 꼭 데이터베이스를
// 연결한 후 서버를 실행시키시길 바랍니다.
createConnection()
.then(() => {
console.log("DB CONNECTION!");
app.listen(3000, () => {
console.log("Server Starting... 3000");
});
})
.catch((error) => {
console.log(error);
});
- index.ts(다른 연결방법)
import { createConnection } from "typeorm";
import connectionOptions from "./ormconfig";
createConnection(connectionOptions)
.then(() => {
console.log("DB CONNECTION!");
app.listen(3000, () => {
console.log("Server Starting... 3000");
});
})
.catch((error) => {
console.log(error);
});
여기서 불러온 ormconfig 는 json 파일이 아닙니다
(🙅🏻♂️애초에 json 파일은 불러올 수 없습니다!)
ormconfig.ts 파일이며, 기존 json 형태로 되어있던 객체를 export 할 수 있도록 수정했고
typeorm 모듈의 ConnectionOptions 라는 type alias(즉, 커스텀된 타입입니다) 를 불러온 후
ormconfig.ts 의 옵션객체의 타입으로 지정해주었습니다
그 후, createConnection 메소드의 인자값으로 주었습니다
- 참고로 ormconfig.ts 에서 옵션객체의 타입에 ConnectionOptions 타입을 지정해주지 않으면 createConnection 에서 에러가 납니다
에러의 내용은 아래와 같습니다
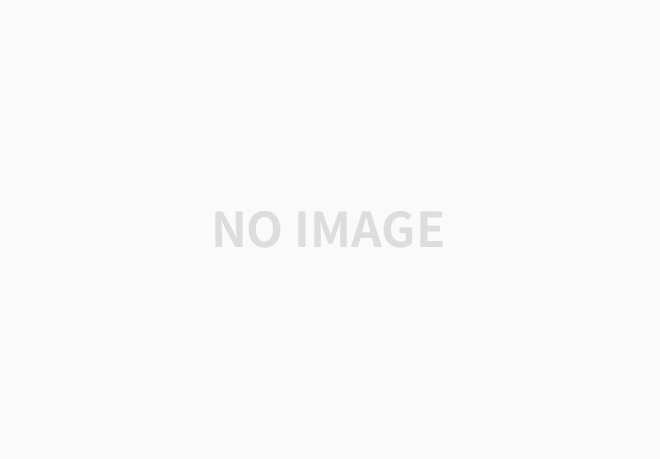
😅 한마디로, 인자값으로 지정된 타입(type)이 있는데, 해당 타입을 지정해주지 않았다는 얘기입니다
조금더 풀어서 말하자면, createConnection 메소드를 구현한 원본코드에서 createConnection 메소드의 매개변수의 타입을 지정했는데, 그것이 ConnectionOptions 라는 타입인 것 같습니다
ConnectionOptions 에 대한 더 자세한 내용은 아래의 링크를 참고하세요!
"connection/ConnectionOptions" | typeorm
typeorm.delightful.studio
- 데이터베이스 종류별 ConnectionOptions 정리
typeorm/typeorm
ORM for TypeScript and JavaScript (ES7, ES6, ES5). Supports MySQL, PostgreSQL, MariaDB, SQLite, MS SQL Server, Oracle, SAP Hana, WebSQL databases. Works in NodeJS, Browser, Ionic, Cordova and Elect...
github.com
- 아래는 ormconfig.ts 의 전체 코드입니다!
import dotenv from "dotenv";
import { ConnectionOptions } from "typeorm";
dotenv.config();
const ormconfig: ConnectionOptions = {
type: "mysql",
host: process.env.DB_HOST,
port: Number(process.env.DB_PORT),
username: process.env.DB_USERNAME,
password: process.env.DB_PASSWORD,
database: process.env.DB_DATABASENAME,
synchronize: true,
logging: false,
entities: ["dist/entity/**/*.js"],
migrations: ["src/migration/**/*.ts"],
subscribers: ["src/subscriber/**/*.ts"],
cli: {
entitiesDir: "src/entity",
migrationsDir: "src/migration",
subscribersDir: "src/subscriber",
},
};
export default ormconfig;
- index.ts (전체 코드)
import express, { Request, Response, NextFunction } from "express";
import cors from "cors";
import userRouter from "./routes/user";
import { createConnection } from "typeorm";
import connectionOptions from "./ormconfig";
const app = express();
app.use(express.json());
app.use(express.urlencoded({ extended: false }));
app.use(
cors({
origin: true,
methods: ["GET", "POST", "OPTIONS", "PUT", "DELETE"],
credentials: true,
})
);
app.use("/user", userRouter);
app.get("/", (req: Request, res: Response) => {
res.send("Hello!");
});
createConnection(connectionOptions)
.then(() => {
console.log("DB CONNECTION!");
app.listen(3000, () => {
console.log("Server Starting... 3000");
});
})
.catch((error) => {
console.log(error);
});
2. typeorm 엔티티(entity) 확인
이제 typeorm 을 사용해서 테이블을 조작해보기전에
엔티티(entity) 코드를 살펴보겠습니다
참고로, typeorm 의 엔티티는 모델과 같은 뜻입니다
🎯 엔티티 코드가 작성되어 있으면 위의 createConnection 메소드를 통해 데이터베이스를 연결하면 자동으로 엔티티에 작성된 칼럼들을 가지고 있는 테이블이 생성됩니다.
- entity/User.ts
import { BaseEntity, Entity, PrimaryGeneratedColumn, Column } from "typeorm";
@Entity()
// BaseEntity 를 상속한 이유는 해당 엔티티(User) 에서
// save, remove 등의 메소드를 사용하기 위함입니다.
export class User extends BaseEntity {
@PrimaryGeneratedColumn()
id: number;
@Column()
name: string;
@Column()
location: string;
}
3. typeorm CRUD 기능 구현
typeorm 을 통해 CRUD 기능을 구현한 코드는 아래와 같습니다
😅 조회와, 생성만 구현해보았습니다
사용자 전체 조회
- allUserSearch.ts
import { Request, Response } from "express";
import { getRepository } from "typeorm";
import { User } from "../../entity/User";
const allUserSearch = async (req: Request, res: Response) => {
try {
// getRepository 메소드를 통한 엔티티 인스턴스 생성
const users: User[] = await getRepository(User).find();
res.status(200).send({ users });
} catch (e) {
res.status(400).json({ message: e.message });
throw new Error(e);
}
};
export default allUserSearch;
사용자 생성
- createUser.ts
import express, { Request, Response } from "express";
import { getRepository } from "typeorm";
import { User } from "../../entity/User";
const insertUser = (req: Request, res: Response) => {
const user: User = new User();
console.log(req.body);
user.name = req.body.name;
user.location = req.body.location;
try {
user.save();
res.status(201).send("new User!");
} catch (e) {
throw new Error(e);
}
};
export default insertUser;
참고
- 엔티티 코드의 typeorm 을 통한 테이블 조작 및 몇가지 데코레이터에 대한 설명
Typescript : mysql을 typeorm 으로 쉽게 - 사용편
설치방법https://blog.naver.com/psj9102/221427742326안녕하십니까. NoDe 입니다.이번엔 typeorm을 사...
blog.naver.com
- typeorm 엔티티 인스턴스 생성 및 저장, typeorm 엔티티 관계, typeorm 마이그레이션
하나의 비디오에서 처음부터 MySQL을 사용하여 Node.js에서 TypeOrm 배우기
비디오 튜토리얼 Typeorm 소개 TypeORM은 NodeJS, Browser, Cordova, PhoneGap, Ionic, React Native, NativeScript, Expo 및 Electron 플랫폼에서 실행할 수 있으며 TypeScript 및 JavaScript (ES5, ES6, ES7, ES8)와 함께 사용할 수있는 O
ichi.pro